Using data from models
Loading a record into a form
When creating a form from a model, you can immediately load values from the database into the form for further editing. This feature is used, for example, when editing data in personal accounts, users on the site.
class Clients extends Model
{
protected $name = 'Clients';
protected $model_elements = [
['Name', 'char', 'name', ['required' => true]],
['E-mail', 'email', 'email', ['required' => true, 'unique' => true]],
['Password', 'password', 'password', ['required' => true]],
['City', 'enum', 'city', ['foreign_key' => 'Cities', 'empty_value' => true]]
];
}
$client = $mv -> clients -> find(93);
$form = new Form('Clients', $client -> id);
//Loading data into the form
$form -> loadRecord();
//Only selected fields can be loaded
$form -> loadRecord(['name', 'city']);
echo $form -> display();
Foreign key filtering
A form built on one model can use data from another model in enum and many_to_many fields. There may be situations when not all foreign key options need to be shown in the form and they need to be filtered or sorted. Filtering parameters are described in the Query builder section.
An example of a user registration form with a city selection.
//Model class for the 'city' field of the client
class Cities extends Model
{
protected $name = 'Cities';
protected $model_elements = [
['Activate','bool', 'active', ['on_create' => true]],
['Name', 'char', 'name', ['required' => true]],
['Country', 'enum', 'country', ['foreign_key' => 'Countries']]
];
}
//In the template file, we show the filtered cities in the form
$form = new Form('Clients');
$form -> filterValuesList('city', ['active' => 1, 'order->asc' => 'name']);
echo $form -> display();
Previous section
Working with form data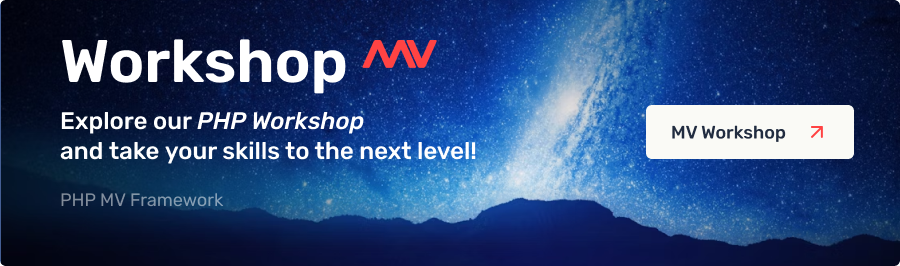