Filtration
When displaying data lists from models, filtering is performed based on parameters from the Query builder section. To run filters on model fields, the Filter class and its object, accessible from the model object, are used.
class Furniture extends Model
{
protected $name = 'Furniture';
protected $model_elements = [
['Active', 'bool', 'active', ['on_create' => true]],
['Images', 'multi_images', 'images'],
['Description', 'text', 'description'],
['Price', 'int', 'price'],
['Room area', 'int', 'square'],
['Location', 'enum', 'location', ['empty_value' => true,
'values_list' => [
'bedroom' => 'In the bedroom',
'livingroom' => 'In the living room',
'childrenroom' => 'In the children room',
'corridor' => 'In the corridor'
]]]
];
}
We are interested in the price, square and location fields, by which we will filter the products. In the template file, we will create an object of the Filter class, which can accept GET parameters with filter values in the form ‘price-from=12000&square-to=50&location=livingroom’.
When the Filter object is launched, the search for the required GET parameters is launched automatically.
<?
//Template file
$mv -> furniture -> runFilter(['price', 'square', 'location']);
?>
<form method='get' action='<? echo $mv -> root_path; ?>furniture'>
<? echo $mv -> furniture -> filter -> display(); ?>
<button>Search</button>
</form>
In the model, in the method for outputting the list of products, we add filter parameters.
class Furniture extends Model
{
...
public function display()
{
//Array of conditions of the specified filters
$conditions = $this -> filter -> getConditions();
//Additional conditions
$conditions['active'] = 1;
$rows = $this -> select($conditions);
...
}
}
When displaying filters in templates, you can use various methods and parameters.
//Running the filter object for the model
$mv -> furniture -> runFilter(['price', 'square', 'location']);
//Outputting fields of all running filters
echo $mv -> furniture -> filter -> display();
//Outputting selected filters in the desired sequence
echo $mv -> furniture -> filter -> display(['location', 'price']);
//Outputting one filter
echo $mv -> furniture -> filter -> display('square');
Filter object methods
- display() - outputs HTML code for filter fields or a single field
- displayCheckbox($field) - outputs HTML code for fields of type bool, image, file, multi_images as a checkbox element (by default, the select tag is output with options yes, no, not set). In case of checkbox, the value 'no' is not defined
- getConditions() - returns an array of values of the passed filters, in the Query builder format
- getUrlParams() - returns a list of GET parameters of filter values for the URL
- addUrlParams($path) - adds the values (GET parameters) of the installed filters to the passed URL
- hasParams() - checks if at least one filter is installed, if so, returns true
- getValue($field, [$condition]) - returns the filter value if it is passed in GET, the second optional parameter is needed for fields of the date, date_time, int, type, float, order, in which values are passed as intervals. The parameter accepts the values 'from' and 'to'.
- setValue($field, $value, [$condition]) - allows you to insert a value for the filter, accepts the field name and value as arguments. The optional parameter is needed to pass parameters for interval filters, similar to the previous method.
- addFilter($caption, $type, $name, [$extra_params]) - addsa new filter to the filter object, arguments are similar to data types
- removeFilter($field) - removes a filter by field name
- setCaption($field, $caption) - sets the name (caption) of a field in the filter list
- setEnumEmptyValueTitle($field, $title) - for enum type fields, you can change the caption of an empty value (used in cases where you need to put its name in the select tag itself). For this model element, the 'empty_value' => option must initially be set. true
- displaySingleField($field) - for date, date_time, int, float, order fields, 2 input windows are displayed by default to set the range of values from and to. If the interval is not needed and only one form element needs to be displayed, then this method should be used.
- filterValuesList($field, $params) - for enum fields with a foreign key, parent and many_to_many, it is possible to filter the list of values using parameters in the Query builder format, $field is the field name, $params is an array of parameters for filtering and/or ordering.
- addOptionToValuesList($field, $value, $title) - for enum and parent fields, allows you to add a value to the list of options
- setDisplaySingleField($field) - for date, date_time, int, float, order fields, sets the option that will display the field as a single text field, rather than an interval of two fields. Also, the filter will accept a value as a single GET parameter, instead of interval values 'from' and 'to'.
- setDisplayCheckbox($field) - for fields of type bool, image, file, multi_images sets the display option as a checkbox element, as a result of calling the display() function
- setDisplayEnumRadio($field, $columns) - for fields of type enum sets the option to output html code as a table with radio buttons, the $columns parameter sets the number of columns in the table.
- setDisplayEnumCheckboxes($field, $columns, [$empty_checkbox]) - for a field of type enum sets the ability to display a list of values as a table with checkboxes. Parameters $field - field name, $columns - number of columns in the table, $empty_checkbox - optional parameter indicating whether to display the checkbox with an empty value first in the list (for example, for manufacturers 'All manufacturers'). Outputs an html table with checkbox fields. This way you can organize multiple selection. The filter for this field in this case will return a value like '2,7,24'.
- setDisplayCheckboxTable($field, $columns) - for many_to_many fields sets the display as a table with checkboxes similar to the previous method
- setManyToManyEmptyValueTitle($field, $title) - for many_to_many fields sets the caption for the empty value when the filter is displayed by the select tag.
//Getting filter values
$mv -> furniture -> filter -> getValue('location');
$mv -> furniture -> filter -> getValue('price', 'from');
$mv -> furniture -> filter -> getValue('price', 'to');
//Adding filter parameters to the URL
$path = $mv -> root_path.'search/?page=3';
$path = $mv -> furniture -> filter -> addUrlParams($path);
//If at least one filter is set
if($mv -> furniture -> filter -> hasParams())
{
...
}
//Setting a label for an empty value of the 'enum' field
$mv -> furniture -> filter -> setEnumEmptyValueTitle('location', 'Select location');
echo $mv -> furniture -> filter -> display('location');
//Make multiple selection possible for the 'Location' field
$mv -> furniture -> filter -> setDisplayEnumCheckboxes('location', 2);
//Set the field to be displayed as radio buttons in 3 columns
$mv -> furniture -> filter -> setDisplayRadio('location', 3);
//The 'Active' field will be displayed as a checkbox
$mv -> furniture -> filter -> setDisplayCheckbox('active');
//The price field will be displayed as a single field for setting a value without an interval
$mv -> furniture -> filter -> setDisplaySingleField('price');
//We display all filter fields with all specified options
echo $mv -> furniture -> filter -> display();
Notes and recommendations
- As a result of calling the getConditions() method, a set of conditions is returned, which the query builder will connect with each other via AND, and if multiple selection is specified in the form of a table with checkboxes for enum and many_to_many fields, then a construction of the form 'field->in' => '8,25,87' will be returned, which indicates a connection for this field via 'OR'.
- Fields of the date, date_time, int, float, order types are filtered by intervals 'from' and 'to'. When calling the display() method for such a field, 2 text fields of the form are returned to specify the interval ('from', 'to'). For example, in the Furniture model, fields with the names 'price-from' and 'price-to' will be displayed for the price field. If you need to display one common field without intervals, then the displaySingleField() method is used.
- A field of the Many to many type will initially be displayed as a result of calling the display() method as a select element with the ability to select one value. If the field is set to display as checkboxes using the setDisplayCheckboxTable($field, $columns) method, then a list of checkboxes with the ability to select multiple values will be displayed.
- For fields of the image, file, multi_images type, the getConditions() method returns the 'field->like' => '.', which in a SQL query will be a check for the presence of a file path in a cell of the SQL table, but if you need to check for the presence of a real file on the disk, you need to use PHP functions for working with the file system.
Previous section
Sorting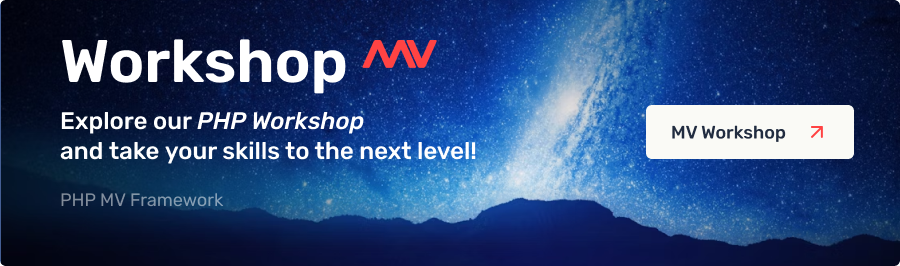