Redirects and http
The $mv object has methods for reloading and redirecting between application pages.
Reloading the current page
This method reloads the current page and allows you to add additional parameters to the URL. The original GET parameters are removed. If no parameters are passed to the method, the current page is loaded without the old GET parameters.
//Template file in the views folder
//Simple reload of the current URL
$mv -> reload();
//If the form is filled in without errors, reload the current page
//and add the GET parameter
if($form -> isValid())
{
...
$mv -> reload('?done');
}
Redirect to another url
This method is used to go to another page. If it is called without parameters, it goes to the main page. You can add both parts of links and GET parameters.
//Go to URL
$mv -> redirect('/catalog/sale');
//If the user is not authorized, go to the login page
if(!$mv -> clients -> checkAuthorization())
$mv -> redirect('/login');
//If the registration form is filled in correctly, we make the transition
//and if we possibly need to redirect the user directly to checkout
if($form -> isValid())
{
...
$path = isset($_GET['from-order']) ? '/order' : '/registration/complete';
$mv -> redirect($path);
}
Error 404
If the requested page cannot be defined in the URL configurations (for more details, see General principles of templates), then the MV displays its own 404 error page. The included file is located in the views folder and is initially called view-404.php.
The display404() method can also take a parameter. If this parameter is positive, then no transition will occur.
//Simple call to 404 error
$mv -> display404();
//If the product is not found, then show 404 error
//If the product is retrieved and the $content variable is an object,
//then continue displaying the page
$content = $mv -> products -> find(['url' => 'very-interesting-book', 'active' => 1]);
$mv -> display404($content);
Http class methods
For ease of development, there are a number of methods in the Http class.
//Is the current request a GET method
Http::isGetRequest();
//Is the current request a POST method
Http::isPostRequest();
//Is the current request an AJAX request
//$method - optional parameter of the request type (GET/POST)
//$exit - optional parameter, whether to terminate the script
//if the request is not AJAX (default false)
Http::isAjaxRequest(string $method, bool $exit);
//Get data from php://input
//$as_array - whether to decode JSON into an array, default false
Http::getRawRequestData(bool $as_array);
//Send JSON response, $json is an array
Http::responseJson(array $json);
//Send XML response, $xml is an XML string
Http::responseXml(string $xml);
//Send text response, $text is a string
Http::responseText(string $text);
//If the current connection is https
Http::isHttps();
//If the current host is local
Http::isLocalHost();
//Set cookie
Http::setCookie(string $key, string $value [, array $params = []]);
Previous section
Date and time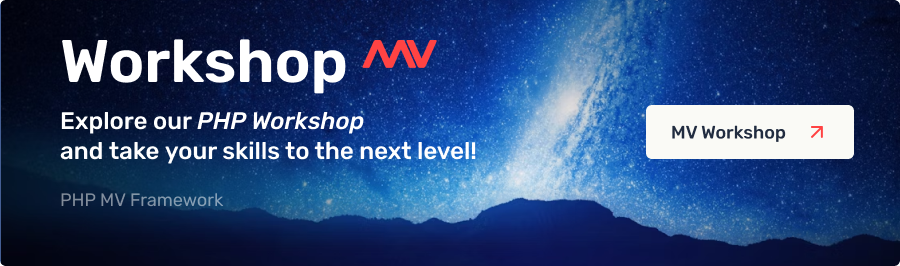