Working with form data
After validating the form data, you can extract the field values for further use.
//The value of a specific field
$staff = $form -> getValue('staff');
//Similarly, using getter
$staff = $form -> staff;
//The title of the enum field (not the key)
$form -> getEnumTitle('gender');
//All form values, returns the associated array
$form -> all();
//Selected form values, returns the associated array
$form -> all(['company', 'about', 'staff']);
//Passing form values to the Record object
$values = $form -> all(['company', 'type', 'email']);
$record -> setValues($values);
$record -> create();
//Or in one line
$record -> setValues($form -> all()) -> update();
//Creating a list of all fields for sending an email message
$message = $form -> composeMessage();
//Creating a list of selective fields in the desired sequence for an email message
$message = $form -> composeMessage(['company', 'about', 'email']);
A complete example of processing form data
Let's assume there is a URL /question that should open a contact form. Let's create a template and write the template file in the routes.
//config/routes.php file
'/question' => 'view-question.php',
Also create a new page in the pages module with the Link field equal to 'question'.
The contents of the views/view-question.php file are shown below.
<?
$content = $mv -> pages -> find(['url' => 'question', 'active' => 1]);
$mv -> display404($content);
$fields = [
['Name', 'char', 'name', ['required' => true]],
['Email', 'email', 'email', ['required' => true]],
['Message', 'text', 'message', ['required' => true]],
['Files', 'file', 'files', ['files_folder' => 'files_questions', 'multiple' => 3]],
['Security code', 'char', 'captcha', [
'required' => true,
'captcha' => 'extra/captcha-simple',
'session_key' => 'captcha']]
];
$form = new Form($fields);
$form -> useTokenCSRF();
$form -> submit() -> validate();
if($form -> isSubmitted() && $form -> isValid())
{
$message = $form -> composeMessage(['name', 'email', 'files', 'message']);
Email::send('hello@world.com', 'Question from site', $message);
$mv -> reload('?sent');
}
include $mv -> views_path.'main-header.php';
?>
<div id="content">
<h1><? echo $content -> name; ?></h1>
<?
echo $form -> displayErrors();
if(isset($_GET['sent'])):
?>
<div class="success">Your message has been sent successfully.</div>
<? else: ?>
<form method="post" enctype="multipart/form-data">
<? echo $form -> display(); ?>
<div class="buttons">
<? echo $form -> displayTokenCSRF(); ?>
<button>Submit</button>
</div>
</form>
<? endif; ?>
</div>
<?
include $mv -> views_path.'main-footer.php';
?>
Previous section
Form security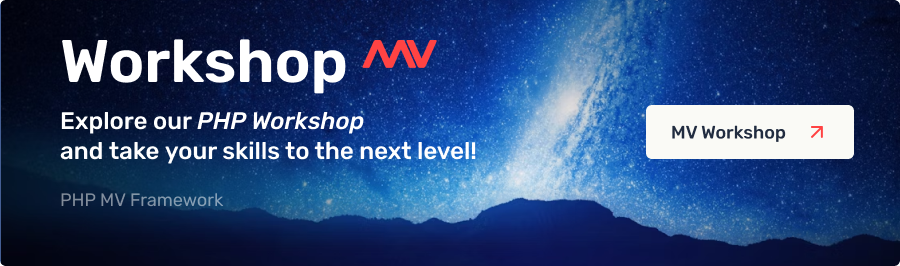