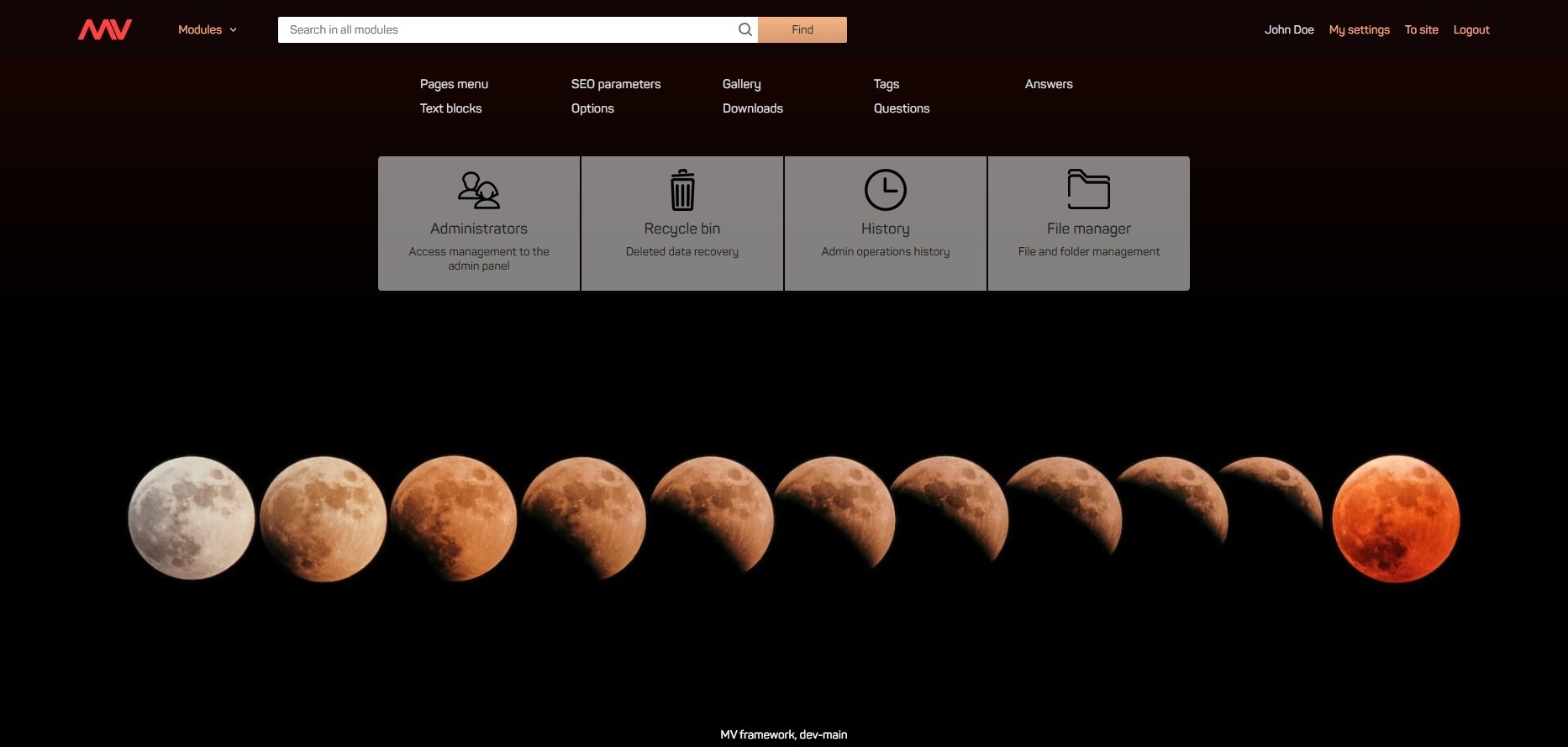
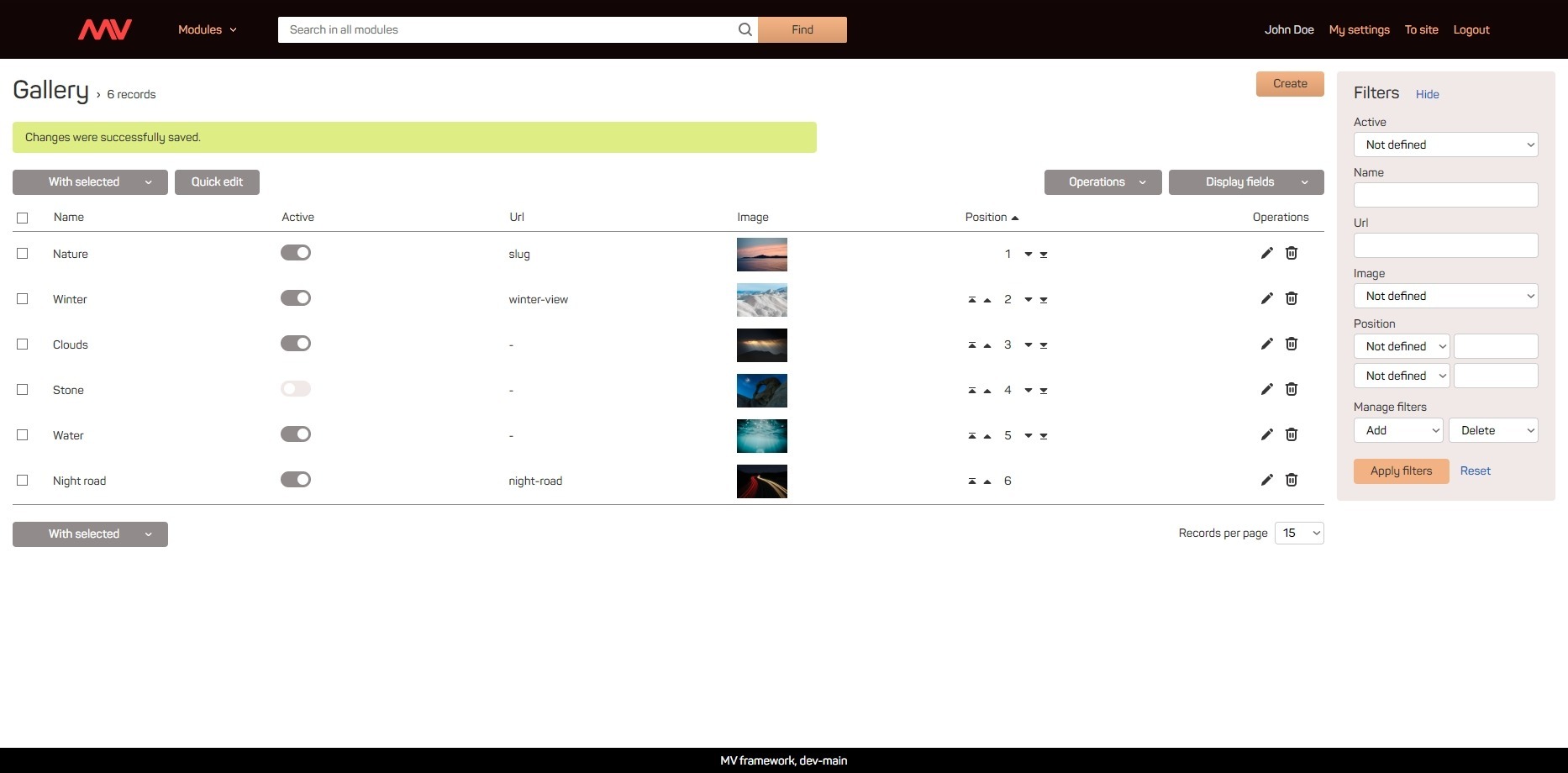
Main advantages
- Object-oriented approach and principles MVC
- Automatic generation of admin panel
- Fast add and update of modules
- ORM and ready functional blocks pagination, sorting, filtration
- Visual editor, batch image upload and much more
Brief overview of MV
Fast and easy creation of data models
class Articles extends Model
{
protected $name = 'Articles';
protected $model_elements = [
['Active', 'bool', 'active', ['on_create' => true]],
['Name', 'char', 'name', ['required' => true]],
['Date', 'date', 'date', ['required' => true]],
['Content', 'text', 'content', ['rich_text' => true]],
['Images', 'multi_images', 'images']
];
}
Simplified and clear routing
'/' => 'view-index.php',
'e404' => 'view-404.php',
'fallback' => 'view-default.php',
'/articles' => 'modules/view-articles.php',
'/articles/*' => 'modules/view-article-details.php',
'/ajax/question' => 'ajax/ask-question.php'
Native PHP templates for generating HTML markup
<div id='inner'>
<div class='date'><? echo I18n::formatDate($article -> date); ?></div>
<h1><? echo $article -> name; ?></h1>
<div class='text'>
<? echo $article -> content; ?>
</div>
<div class='gallery'>
<? echo $mv -> articles -> displayGallery($article -> images); ?>
</div>
</div>
Simple automatic or manual installation
composer create-project makscraft/mv-framework my_app