Additional features
MV allows additional data processing inside the core methods. To do this, you need to overload the model methods.
Additional data validation in the model
Suppose you need to enter data in the percentage format '12%'. We need to check the entered data for compliance with the regular expression pattern.
class Discounts extends Model
{
protected $name = 'Discounts';
protected $name_field = 'amount';
protected $model_elements = [
['Active', 'bool', 'active', ['on_create' => true]],
['Discount amount, %', 'char', 'amount', [
'required' => true,
'help_text' => 'example 10%, 17%, 30%'
]],
['Amount from', 'int', 'summ_from', ['required' => true]],
['Amount to', 'int', 'summ_to', ['zero_allowed' => false]]
];
public function validate()
{
$amount = $this -> elements['amount'] -> getValue();
if($amount && !preg_match('/^[1-9]d?%$/', $amount))
$this -> errors[] = 'The discount amount must be specified as an integer with % at the end.';
return parent::validate();
}
}
Getting and changing model field values
You can get the properties of its fields from the model object, as well as set new properties for the fields.
- getFieldProperty($field, $property) - get the value of the $property property of the $field field
- setFieldProperty($field, $property, $value) - set the value equal to $value of the $property property of the $field field
$value = $mv -> discounts -> getFieldProperty('summ_to', 'zero_allowed');
if( ... )
$mv -> discounts -> setFieldProperty('summ_to', 'zero_allowed', true);
Formatting fields in a table
When displaying a table of model records in the admin panel, the field values can be changed as needed. For this, a special method can be added to the model that can convert the field values for the table.
- processAdminModelTableFields($name, $row) - where $name is the field name, $row is an array of values for all record fields, including id.
class Stock extends Model
{
...
//Add the method to the model and in the column with weights next to the values there will be 'kg'
public function processAdminModelTableFields($name, $row)
{
if($name === 'weight' && $row['weight'] > 0)
return $row['weight'].' kg';
}
}
Previous section
Managing simple models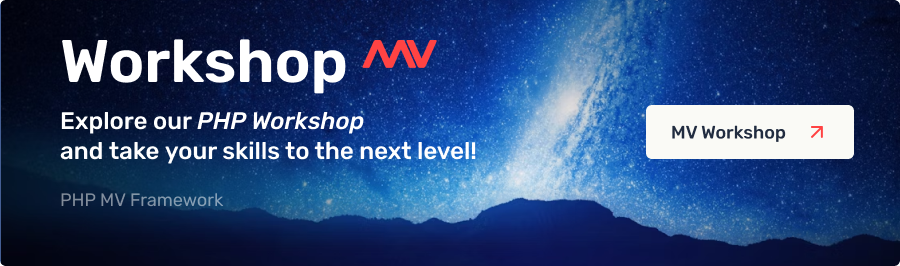