Files and images
There are 3 types of data for storing files in MV:
- file - single file
- image - single image
- multi_images - array of images with comments
The database stores paths to files from the project root without the leading slash. Files of the file type are stored in folders userfiles/models/model_name-files/. All images of models are located in folders of the form userfiles/models/model_name-images/. When uploading to the server, in case of duplicate names, each file is assigned a unique number.
Reduced copies of images are created in subfolders, with the subfolder name set automatically based on the dimensions and name of the current model, for example userfiles/models/gallery-images/gallery_70x70/name-of-image.jpg.
Display files for downloading and images
displayFileLink($field [, $link_text, $no_file_text]) - returns a link to a file for downloading
$link_text - the name of the file to insert into the link (if absent, the name of the file itself will be supplied)
$no_file_text - if the file is absent and this parameter is specified, this text will be displayed- displayImage($field, [, $extra_params]) - returns the img tag with this image
Resizing and cropping images
- cropImage($image, $width, $height [, $extra_params]) - reducing the image to the specified dimensions with cropping
- resizeImage($image, $width, $height [, $extra_params]) - proportionally reducing the image without cropping
The cropImage() and resizeImage() methods can be called from a model object or from a Record class object, and they create a folder for reduced copies based on the file's belonging to a specific model.
Additional parameters can be passed as an associated array $extra_params. Possible parameter options (keys): 'alt-text', 'no-image-text', 'title', 'css-class'. None of the parameters are required. When passing a parameter, it will be added to the img tag.
Working with an array of images
- extractImages($field) or asArrays($field) - converting to an array of images
- getFirstImage($field) - extracting the first image from the array
Let's create a model and extract the necessary data in the templates.
class Products extends Model
{
protected $name = 'Products';
protected $model_elements = [
['Name', 'char', 'name', ['required' => true]],
['Main image', 'image', 'main_image'],
['Additional images', 'multi_images', 'extra_images'],
['Manual', 'file', 'manual'],
['Description', 'text', 'text_description']
];
}
We display a list of all products with images for the general template, file models/products.model.php.
<?
public function display()
{
$rows = $this -> select();
$html = '';
foreach($rows as $row)
{
$url = $this -> root_path.'product/'.$row['id'];
$html .= '<div>';
$html .= '<div class="name"><a href="'.$url.'">'.$row["name"].'</a></div>';
$html .= '<div class="image">';
$html .= $this -> cropImage($row["main_image"], 150, 150, ['alt-text' => $row['name']]).'</div>';
$html .= '<div class="description">';
$html .= Service::cutText($row["text_description"], 200, "...").'</div>';
$html .= '</div>';
}
return $html;
}
We display a list of product instructions that have them, file models/products.model.php.
<?
public function displayManuals()
{
$rows = $this -> select(['manual!=' => '']);
$html = '';
foreach($rows as $row)
{
$html .= '<div class="dowload-file">';
$html .= '<a href="'.Service::addFileRoot($row['manual']).'">'.$row['name'].'</a>';
$html .= '</div>';
}
return $html;
}
On the product page, we display all available data, file views/view-product.php.
<?
$product = $mv -> products -> defineProductPage($mv -> router);
$mv -> display404($product);
include $mv -> views_path.'main-header.php';
?>
<div id="content">
<h1><? echo $product -> name; ?></h1>
<div id="main-picture">
<a class="lightbox" href="<? echo $mv -> root_path.$product -> main_image; ?>">
<? echo $mv -> products -> resizeImage($product -> main_image, 300, 250); ?>
</a>
</div>
<div id="extra-pictures">
<?
foreach($product -> extractImages('extra_images') as $image)
{
echo '<a class="lightbox" href="'.$mv -> root_path.$image['image'].'">';
echo $mv -> products -> cropImage($image, 100, 100).'</a>';
}
?>
</div>
<p><? echo $product -> text_description; ?></p>
<p><? echo $product -> displayFileLink('manual', 'Download instructions'); ?></p>
</div>
<?
include $mv -> views_path.'main-footer.php';
?>
Previous section
Record object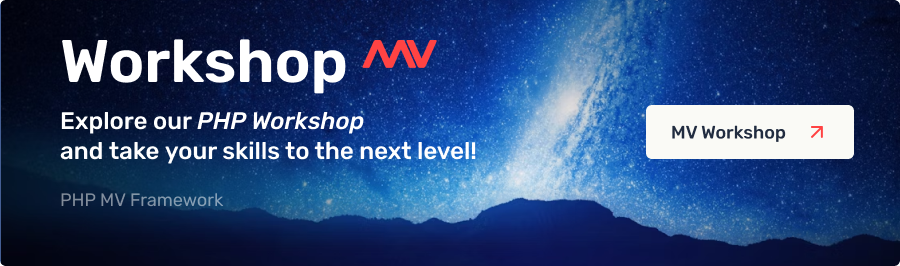