Caching
MV allows you to cache data for quick retrieval. Typically, code fragments that are obtained as a result of a large number of SQL queries and data processing costs are cached. For example, you can cache nested menus, or lists of items that have complex relationships between several tables.
As a result, the code fragment is saved in the database, and only one request is required to retrieve it, instead of many requests spent on its initial generation.
To enable caching, you must set the 'EnableCache' option to true in the config/setup.php file.
Caching for the time period
//Save the string with the 'greeting' key for 100 seconds
$mv -> cache -> save('greeting', 'Hello world!', 100);
//Check cache by key
if(null !== $value = $mv -> cache -> find('greeting'))
{
...
}
Cache is reset for each operation with any of the model records bound to the cache key.
Caching linked to the admin panel
Cache reset can be linked to operations in the admin panel. When a CRUD operation occurs in one or more models, the cache will be reset.
//Save a string with the 'hello' key and clear it on any action with models
$mv -> cache -> save('hello', 'Hello world!', '*');
//Clear the cache on any action with the Pages model
$mv -> cache -> save('hello', 'Hello world!', ['Pages']);
//Clear the cache on any action with several models
$mv -> cache -> save('hello', 'Hello world!', ['Pages', 'News', 'GiftsPromo']);
Caching the method result
Assume that the Catalogs model has a displayLeftCatalogMenu() method that displays a tree of categories and uses many SQL queries for this. It is necessary to cache the method result in the template to speed up page generation. The cache should be cleared only when the Catalogs model changes. Let's modify the code in the template responsible for displaying the catalog.
//Template file
//Before caching
echo $mv -> catalogs -> displayLeftCatalogMenu();
//After applying caching
if(!$mv -> cache -> findAndDisplay('catalog-menu'))
echo $mv -> cache -> save('catalog-menu',
$mv -> catalogs -> displayLeftCatalogMenu(),
['Catalogs']);
If there is a template fragment that is generated from several models, then it is necessary to cache this code fragment and clear it when data changes in any of the participating models.
//Linking cache clearing to actions in the admin panel in several models
if(!$mv -> cache -> findAndDisplay('something'))
echo $mv -> cache -> save('something',
$mv -> pages -> displaySomething(),
['Pages', 'Catalogs', 'News']);
Media files caching
MV can compress a large number of CSS and JS files into one, significantly reducing the number of requests to the server from the browser.
//File views/main-header.php before caching
<link rel="stylesheet" type="text/css" href="<? echo $mv -> media_path; ?>css/style.css" />
<link rel="stylesheet" type="text/css" href="<? echo $mv -> media_path; ?>css/catalog.css" />
<script type="text/javascript" src="<? echo $mv -> media_path; ?>js/jquery.js"></script>
<script type="text/javascript" src="<? echo $mv -> media_path; ?>js/utils.js"></script>
//After caching
<?
CacheMedia::instance();
CacheMedia::addCssFile(['style.css', 'сatalog.css']);
CacheMedia::addJavaScriptFile(['jquery.js', 'utils.js']);
if(...)
CacheMedia::addCssFile('style-special.css');
//This condition is not mandatory.
if(Http::isLocalHost())
echo CacheMedia::getInitialFiles();
else
echo CacheMedia::getAllCache();
?>
If any template includes additional files in the media cache, they must be added before including the main-header.php file.
CacheMedia :: addJavaScriptFile([
'request.js',
'media/jquery-ui/jquery-ui.min.js'
]);
CacheMedia :: addCssFile([
'media/jquery-ui/jquery-ui.min.css',
'media/jquery-ui/jquery-ui.structure.min.css',
'media/jquery-ui/jquery-ui.theme.min.css'
]);
include $mv -> views_path.'main-header.php';
Notes
- You can evaluate the effectiveness of caching by the number of SQL queries and the page generation time. For this, the debug panel is used. To display it, set the ‘DebugPanel’ setting to true in the config/setup.php file. The panel appears at the bottom of the page.
- Caching data is stored in the database in the cache and cache_clean tables, which can be cleared at any time without affecting the project. You can also reset the cache using the static Cache::cleanAll() method.
- The $mv -> cache object has the cleanByKey($key) and cleanByModel($model) methods to clean the cache by the key or name of the model it is assigned to.
- The 'EnableCache' option value set to false completely stops saving and retrieving data from the cache.
Previous section
Plugins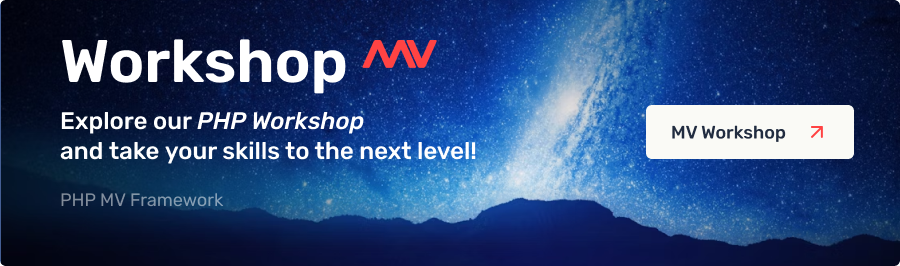