AJAX
To add AJAX functionality to MV, we recommend following these rules:
- If the project is deployed in a subdirectory, add the path from the root to the project to JavaScript.
- Create a route with a readable and understandable URL.
- In the template to which the URL will come, use the methods of the Http class.
Pass the full path to the project to JavaScript in the views/main-header.php file, add a line with the path.
<script type="text/javascript"> const rootPath = '<? echo $mv -> root_path; ?>'; </script>
Add a route and create the required template file to which the AJAX request will go.
//config/routes.php file
'/ajax/form/feedback' => 'ajax/feedback-form.php'
In the JavaScript file, add the code for sending an AJAX request, for example, to JQuery.
$.ajax(
{
type: 'POST',
dataType: 'json',
url: rootPath + 'ajax/form/feedback',
data: $('#feedback-form').serialize(),
success: function(data)
{
//Response processing (for form)
if(data.valid)
{
...
}
else
{
//Array form errors
data.errors.fields
//Single html with form errors
data.errors.all
}
}
});
In the template file, we check the request and prepare a response in JSON or HTML form if we are generating a fragment of HTML code to insert into the page.
//File views/ajax/feedback-form.php
//First, we check that a POST AJAX request has arrived, if not, then exit
Http::isAjaxRequest('post', true);
//Check for parameters in the request, generate HTML
if(isset($_POST['param']))
{
$html = $mv -> model -> method();
...
}
//Or, for example, we process the form
$form -> submit() -> validate();
//At the very end, we return the response in JSON format
$json = ['success' => true];
Http::responseJson($json);
//Other response formats
Http::responseXml($xml);
Http::responseHtml($html);
Http::responseText($text);
If a form is processed in an AJAX request, it is recommended to return a JSON object with the result of the form's getState() method as a response.
//File views/ajax/feedback-form.php
Http::isAjaxRequest('post', true);
$form = new Form('Feedback');
$form -> useAjaxTokenCSRF() -> useJqueryToken();
$form -> submit() -> validate();
if($form -> isValid())
{
...
}
Http::responseJson($form -> getState());
If additional data needs to be added to the form state in the response, the addStateValue() method is used.
//File views/ajax/feedback-form.php
if($form -> isValid())
{
...
$form -> addStateValue('link', 'https://...');
}
Http::responseJson($form -> getState());
Previous section
Filtration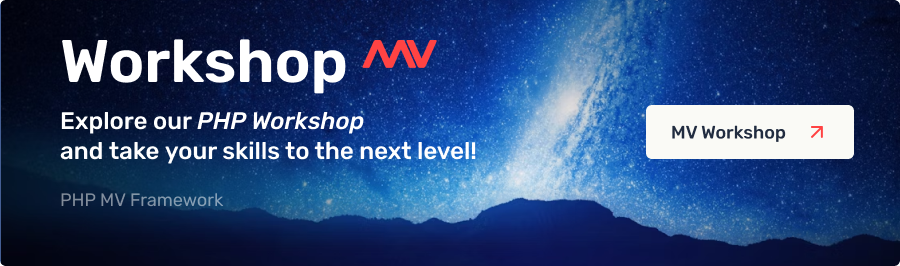