Records management
A record in MV is a single row in the model table. A record consists of fields of different types. For operations on table records, a Record class object is used, which manages one record in the table.
When working with the Record object, the following features must be taken into account.
- During CRUD operations, event methods of the model such as beforeCreate, afterCreate are not launched.
- The Record class object does not save versions and does not check fields for editing restrictions.
- When deleting via the admin panel, the record is placed in the recycle bin, and when using the Record class, it is permanently deleted.
- Methods for working with records affect only those table fields that are declared in the model in the $model_elements array. Theoretically, a database table may have additional fields, which can be worked with via Direct queries to the database.
Let's consider record management using the example of a model of stories that users add to the site.
class Stories extends Model
{
protected $name = 'Stories';
protected $model_elements = [
['Acive', 'bool', 'active'],
['Date', 'date_time', 'date', ['required' => true]],
['Title', 'char', 'name', ['required' => true]],
['Topic', 'enum', 'theme', ['values_list' => [
'family' => 'Family',
'home' => 'Home',
'travel' => 'Travel']]],
['Content', 'text', 'content', ['required' => true]]
];
}
Creating a record
//Create an empty record
$story = $mv -> stories -> getEmptyRecord();
//Enter field data
$story -> active = 1;
$story -> name = 'How to mow the lawn';
$story -> theme = 'home';
$story -> date = I18n::getCurrentDateTime();
$story -> content = 'Text';
//Save to the database
$story -> create();
//Data for fields can also be obtained from an array or form
$story -> setValues([
'active' => 1,
'name' => 'Story name',
'content' => 'Content'
]);
//Passing an array of data from the form
$story -> setValues($form -> getAllValues()) -> create();
//The create() method returns the id of the created record,
//which can be used later
$id = $story -> create();
Editing a record
//Retrieving a record and changing field values
$story = $mv -> stories -> find(75);
$story -> active = 0
$story -> name = 'How to mow the lawn in autumn';
$story -> update();
//You can also get data from an array or form
$story -> setValues($form -> getAllValues(['name', 'content'])) -> update();
$story -> setValues(['active' => 1, 'theme' => 'family']) -> update();
//If you don't know in advance whether the required record exists,
//you can use the following methods
$story = $mv -> stories -> findRecordOrGetEmpty(['name' => 'How to mow the lawn']);
$story -> name = 'How to mow the lawn';
$story -> active = 1;
//Create or update the record (if the record already exists)
$story -> save();
Deleting a record
//The record is deleted without being placed in the trash
$story = $mv -> stories -> find(['name' => 'How to mow the lawn in autumn']);
$story -> delete();
//The record is placed in the trash (can be seen in the admin panel)
$mv -> stories -> setId(35) -> delete();
Bulk editing of records
The updateManyRecords($params, $conditions) method is used, where $params are the parameters to be changed for all records, $conditions are the selection conditions described in the Query builder section.
//Activate all records of the model
$mv -> stories -> updateManyRecords(['active' => 1]);
//Activate all records by a certain attribute
$mv -> stories -> updateManyRecords(['active' => 1], ['theme' => 'home']);
//A more complex variant
$mv -> stories -> updateManyRecords([
'active' => 0,
'content' => ''
], [
'active' => 1,
'theme' => ['travel', 'home'],
'date<=' => '2023-09-25 12:00:00'
]);
Bulk deletion of records
The deleteManyRecords($conditions) method is used, where $conditions are the selection conditions described in the Query builder section.
$mv -> stories -> deleteManyRecords(['active' => 0, 'theme' => 'home']);
Delete all records
The clearTable() method deletes all rows of the table and resets the auto increment parameter for the primary key. If an optional parameter is passed, then not the base table of the model is cleared, but the one whose name is passed in the parameter.
//Clearing the stories table
$mv -> stories -> clearTable();
//Clearing the comments table
$mv -> stories -> clearTable('comments');
Previous section
Group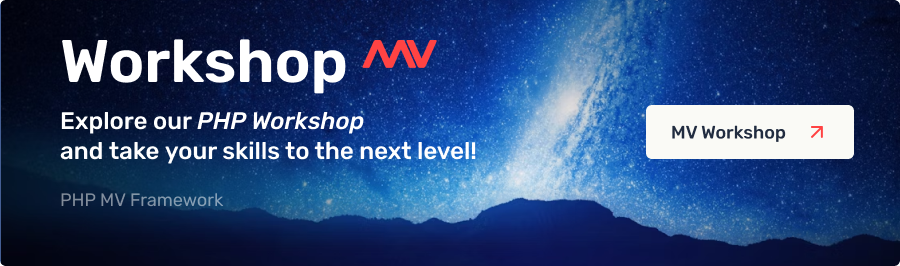