Form security
To ensure the security of forms, MV offers 3 types of verification keys:
- Regular CSRF key for a classic POST request
- AJAX CSRF key for forms running on AJAX
- JQuery key, separately or in addition to the CSRF key
A regular CSRF key is used in forms when a page reload in the browser is expected.
<?
$form = new Form($fields);
$form -> useTokenCSRF();
$form -> submit() -> validate();
?>
<form method="post" enctype="multipart/form-data">
<? echo $form -> display(); ?>
<div class="buttons">
<? echo $form -> displayTokenCSRF(); ?>
<button>Submit</button>
</div>
</form>
A CSRF key for AJAX forms is required when the page does not reload in the browser, and the request is sent to the server in the background with the ‘X-Requested-With’ header.
<?
$form = new Form($fields);
$form -> useAjaxTokenCSRF();
?>
<form method="post" enctype="multipart/form-data">
<? echo $form -> display(); ?>
<div class="buttons">
<? echo Form::displayAjaxTokenCSRF(); ?>
<button type="button">Submit</button>
</div>
</form>
The jQuery key is used in addition to the CSRF key to increase the security of the form, with a classic and ajax request.
//File views/main-header.php
<head>
...
<script type="text/javascript" src="<? echo $mv -> media_path; ?>js/jquery.js"></script>
<? echo Form::displayJqueryToken(); ?>
</head>
//File template with form (ajax or regular)
//For an ajax form you will need a method Form::displayAjaxTokenCSRF()
<?
$form = new Form($fields);
$form -> useTokenCSRF() -> useJqueryToken();
$form -> submit() -> validate();
?>
<form method="post" enctype="multipart/form-data">
<? echo $form -> display(); ?>
<div class="buttons">
<? echo $form -> displayTokenCSRF(); ?>
<button>Submit</button>
</div>
</form>
Using CAPTCHA
To connect CAPTCHA, you need to add an additional field with special parameters to the form. Below is an example of a simple CAPTCHA located in the extra folder. You can connect a more complex captcha at your discretion.
<?
$form = new Form($fields);
$form -> addField(['Security code', 'char', 'captcha', [
'captcha' => 'extra/captcha-simple',
'session_key' => 'captcha'
]];
$form -> addRule('captcha', 'required', true, 'You must enter the verification code.');
?>
<form method="post" enctype="multipart/form-data">
<? echo $form -> display(); ?>
</form>
Previous section
Validating form fields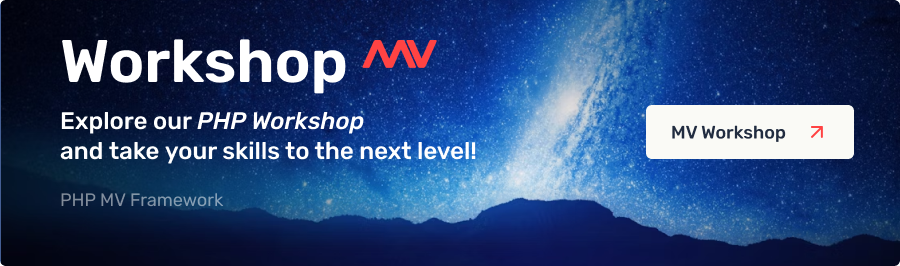