Setting up form fields
Let's create a form and use examples to display HTML in a template and change the parameters of its fields.
$fields = [
['Your name', 'char', 'name', ['required' => true],
['Gender', 'enum', 'gender', ['values_list' => ['Male', 'Female']]],
['Email', 'email', 'email'],
['Receive news', 'bool', 'news_subscribe'],
['Additional information', 'text', 'about', ['help_text' => 'Tell us about yourself']]
];
$form = new Form($fields);
//Output all form fields, by default displayed in div tags
echo $form -> display();
//Output two fields in div tags
echo $form -> display(['name', 'email']);
//Output all form fields, as a table
echo $form -> display('*', 'table');
//Output all form fields (inputs only)
echo $form -> display('*', 'inputs');
//Output selected form fields (inputs only)
echo $form -> display(['name', 'about', 'email'], 'inputs');
//Output html code for a separate form field (without name)
echo $form -> displayFieldHtml('gender');
//Output the form with errors attached to the fields
echo $form -> setDisplayWithErrors() -> display();
//Add placeholders (names) to the fields
$form -> setNamesAsPlaceholders();
echo $form -> display('*', 'inputs');
Adding / removing a field
$form = new Form($fields);
//Operations on fields are performed before validation and display of form fields
$form -> addField(['Phone Number', 'phone', 'phone_number']);
$form -> removeField('news_subscribe');
...
$form -> submit() -> validate();
...
echo $form -> display();
Changing field parameters
//Make a field required
$form -> setRequired('about');
//Make all fields required
$form -> setRequired('*');
//Make several fields mandatory
$form -> setRequiredFields(['email', 'about']);
//Set the field name
$form -> setCaption('email', 'E-mail');
//Set explanatory text for the field
$form -> setHelpText('news_subscribe', 'The newsletter comes no more than once a month');
//Set additional html parameters for the field (attributes: id, class, title)
$form -> setHtmlParams('about', 'id="about-me" class="text-field" rows="12"');
//Additional html parameters for several fields
$form -> setHtmlParams(['email', 'name'], 'class="text-input"');
//Set placeholder for the field
$form -> setPlaceholder('email', 'Email');
Additional features
//If the values of the enum type field are data from another model
//(foreign key), then they can be filtered
$form -> filterValuesList('city', ['active' => 1, 'order->asc' => 'name']);
//For the date type field, you can set the display as 3 select tags
$form -> setDisplaySelects('date');
//For the enum type field, you can set the display as radio buttons
$form -> setDisplayRadio('gender', 2);
//For enum and many_to_many type fields, display the values as a table with checkboxes
//(2 is the number of table columns)
$form -> setDisplayTable('interests', 2);
Previous section
Creating forms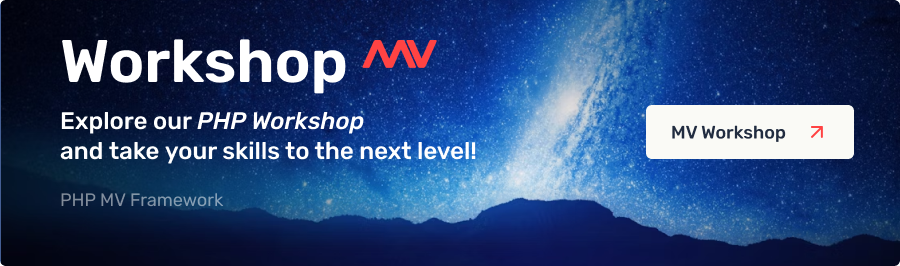