Pagination
To split a long list of records into pages, you can create an object of the Paginator class in any model. To do this, call the runPager($total, $limit) method, where $total is the total number of records, $limit is the number of records per page.
//Template file in the views folder
//Counting active records in the table
$total_active = $mv -> events -> countRecords(['active' => 1]);
//Starting splitting by 10 records per page
$mv -> events -> runPaginator($total_active, 10);
//Now you can view the state of the Paginator object
Debug::pre($mv -> events -> paginator -> getState());
Thus, now we can add pagination to the function for outputting events in the Events model. More details about retrieving records are written in the section Query builder.
public function displayEvents()
{
$rows = $this -> select([
'order->desc' => 'date',
'active' => 1,
'limit->' => $this -> paginator -> getParamsForSelect()
]);
...
}
To display a list of links to other pages, use the display() method of the Paginator class.
//$path - URL to which page numbers will be added ('/news', '/catalog/books')
$path = $mv -> root_path.'events';
echo $mv -> events -> paginator -> display($path);
The final view of the template for displaying a list of events.
<?
$total_active = $mv -> events -> countRecords(['active' => 1]);
$mv -> events -> runPaginator($total_active, 10);
$path = $mv -> root_path.'events';
include $mv -> views_path.'main-header.php';
?>
<div id='content'>
<? echo $mv -> events -> display(); ?>
<div class='paginator'>
<? echo $mv -> events -> paginator -> display($path); ?>
</div>
</div>
<?
include $mv -> views_path.'main-footer.php';
?>
Paginator object methods
- getState() - return an array of pagination parameters
- setLimit($limit), setTotal($total) - set the limit and total number of elements, recalculate intervals
- display($path) - output a list of pagination links, $path is the url to which the GET pagination parameter is added
- displayLimits($limits, $path [, $format = ‘links’]) - output a list of links or options with limit parameters
- hasPages() - checks if there are intervals, number of intervals > 1
- checkPrevNext($type) - checks if there is a next / previous page from the current one, the $type parameter must be 'next' or 'prev'
- displayPrevLink($caption, $path) - displays a link to the previous page if it exists, $caption is the link text, $path is the url to which a parameter like 'page=3' will be added
- displayNextLink($caption, $path) - similar to the previous function, displays a link to the next page
- addUrlParams($path) - adds a GET parameter like 'page=7' (the current page) to the passed URL. Usually used to transfer pagination parameters to other modules, such as a sorter or filter.
- getUrlParams() - returns a GET parameter like 'page=12' (current page), if the total number of pages > 1
Below is an example of displaying a list of pages in a template, taking into account links to the next or previous page.
<?
...
$path = $mv -> root_path.'events';
include $mv -> views_path.'main-header.php';
?>
<div id='content'>
<? echo $mv -> events -> display(); ?>
<? if($mv -> events -> paginator -> hasPages()): ?>
<div class='paginator'>
<span class='title'>Page:</span>
<?
echo $mv -> events -> paginator -> displayPrevLink('previous', $path);
echo $mv -> events -> paginator -> display($path);
echo $mv -> events -> paginator -> displayNextLink('next', $path);
?>
</div>
<? endif; ?>
</div>
<?
include $mv -> views_path.'main-footer.php';
?>
Previous section
Direct queries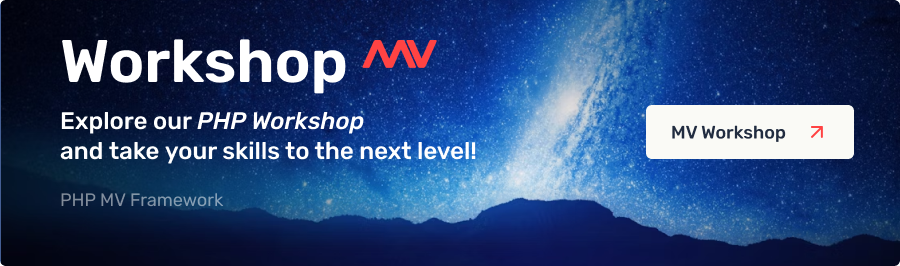