Managing simple models
Simple models have their own list of methods for managing data.
- getValue($field) - extract the field value, described in more detail in the section Data output in the template, or use getter
- setValue($field, $value) - set the field value for subsequent use and/or update
- getEnumTitle($field) - get the name (not the key) of the enum type field
- loadIntoRecord() - extract all fields into the Record class object, the process is described in the section Data output in the template
- update() - update data of a simple model, no new version is written
- combineEmails([$fields]) - takes all fields of the email type and glues them together separated by commas, in the optional parameter you can pass the names of the fields that need to be returned as an array, if the argument is not passed, all filled fields of the email type will be returned.
class Options extends Model_Simple
{
protected $name = 'Settings';
protected $model_elements = [
['Show banner', 'bool', 'banner_active'],
['Administrator email', 'email','admin_email', ['required' => true]],
['Administrator email (orders)', 'email', 'admin_email_orders'),
['Administrator email (feedback)', 'email', 'admin_email_feedback'),
['Phone', 'phone', 'phone'],
['Deal of the day', 'enum', 'promo', ['values_list' => [
'discount' => '10% discount',
'tickets' => 'Concert tickets',
'gift' => 'Special Gift'
]]],
['Advertisement Text', 'text', 'text'],
['Price list', 'file', 'price'],
['Banner', 'image', 'banner'],
['Image gallery', 'multi_images', 'gallery']
];
protected $model_display_params = [
'fields_groups' => [
'Basic settings' => [
'banner_active', 'admin_email', 'admin_email_ordes',
'admin_email_feedback', 'phone', 'promo', 'price', 'banner'
],
'Text' => ['text'],
'Gallery' => ['gallery']
]
];
}
//Fetching data
echo $mv -> options -> phone;
echo $mv -> options -> text;
echo $mv -> options -> getEnumTitle('promo');
//Resizing image
echo $mv -> options -> cropImage('banner', 200, 200);
//Update simple model data
$mv -> options -> setValue('admin_email_feedback', 'user@mail.com')
-> setValue('text', 'Hello!');
-> update();
//Array of images
$images = $mv -> options -> extractImages($mv -> options -> gallery);
//Create a list of email addresses
$recipients = $mv -> options -> combineEmails();
Email::send($recipients, 'Message from the site', 'Message text');
Previous section
Records management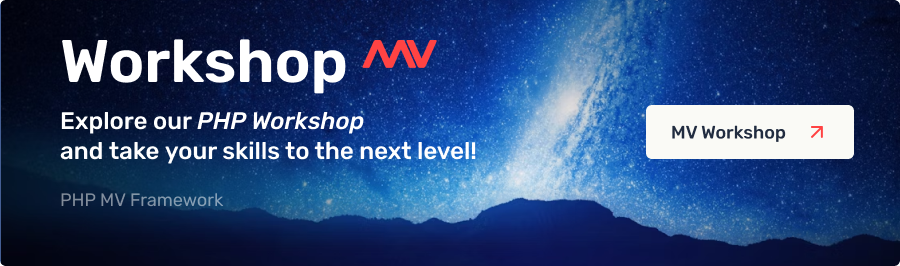