Record object
A record from a table can be retrieved using the find() model method, which returns an object of the Record class. An object of this class provides the ability to access and change the values of the record fields in the table. The $conditions selection parameters are an associated array described in the Query builder section.
For example, let's take a model that describes books.
class Books extends Model
{
protected $name = 'Books';
protected $model_elements = [
['Name', 'char', 'name', ['required' => true]],
['Author', 'enum', 'author', ['foreign_key' => 'Authors']],
['Date published', 'date', 'date'],
['Cover photo', 'image', 'photo'],
['Brief description', 'text', 'description']
];
}
Add a method to the model to display the list of books.
<?
public function display()
{
//All entries in alphabetical order
$rows = $this -> select(['order->desc' => 'name']);
$html = '';
//Create a link to each book
foreach($rows as $row)
$html .= '<li><a href="'.$this -> root_path.'books/'.$row['id'].'">'.$row['name'].'</a></li>';
return $html;
}
After calling the display() method, we will see a list of links on the page with books. Let's create a template file view-book.php to display information about the book.
//File config/routes.php
'/books' => 'view-books-all.php',
'/book/*' => 'view-book.php',
Contents of the file views/view-book.php
<?
//We take the second part of the URL and check that it is numeric
$book_id = $mv -> checkUrlPart(1, 'numeric');
//Extract a record by its id
$book = $mv -> books -> find($book_id);
//If the record is not found, we show a 404 error
$mv -> display404($book);
<?
include $mv -> views_path.'main-header.php';
?>
<div class="content">
<h1><? echo $book -> name; ?></h1>
<h3>Author:<? echo $book -> getEnumTitle('author'); ?></h3>
<div>Date:<? echo I18n::formatDate($book -> date); ?></div>
<? echo $book -> resizeImage('photo', 200, 300); ?>
<h4>Brief description</h4>
<p><? echo nl2br($book -> description); ?></p>
</div>
<?
include $mv -> views_path.'main-footer.php';
?>
Similarly, you can take the newest book from the list or the first book of a specific author.
$book = $mv -> books -> find(['order->desc' => 'date']);
$book = $mv -> books -> find(['order->asc' => 'date','author' => 42]);
How the Record object works
Access to the fields of the retrieved record is carried out as access to the object properties (if the object has this property, its value will be returned).
//Getting a property
echo $book -> name;
echo I18n::formatDate($book -> date);
echo nl2br($book -> description);
//Setting the property value
$book -> name = 'Mobi-Dik';
$book -> description = 'American romantic literature.';
//Updating the record
$book -> update();
//All fields of the record
Debug::pre($book -> all());
//Id of the record 'Herman Melville' in the Authors model table
$book -> author = $mv -> authors -> find(['name' => 'Herman Melville']) -> id;
//Extracting the name (title) of a field of type 'enum'
echo $book -> getEnumTitle('author');
//Resizing and displaying an image
echo $book -> resizeImage('photo', 200, 300);
echo $book -> cropImage('photo', 100, 100);
Other methods
- setValues($values) - pass an array of field values to the Record object
- all() - extract the values of all fields as an associated array
- getEnumTitle($field) - get the name (not the key) of the enum type field
The Record class object has methods for working with files and images, described in the section Files and images:
- resizeImage($field, $width, $height) - compress an image while maintaining proportions
- cropImage($field, $width, $height) - image compression and cropping
- displayImage($field) - output html img tag for image field with original dimensions
- displayFileLink($field) - output link to file for downloading
- asArrays($field) - extracting images of type multi_images
- getFirstImage($field) - extracting the first image from field of type multi_images
Let's assume that the model has field of type multi_images with name 'pictures', and $product is instance of class Record.
//Array with paths to images and comments
$images = $product -> extractImages('pictures');
$images = $product -> asArrays('pictures');
//First image in the array
$image = $product -> getFirstImge('pictures');
Also in the Record class there are CRUD methods described in the section Records management.
Previous section
Output of data in template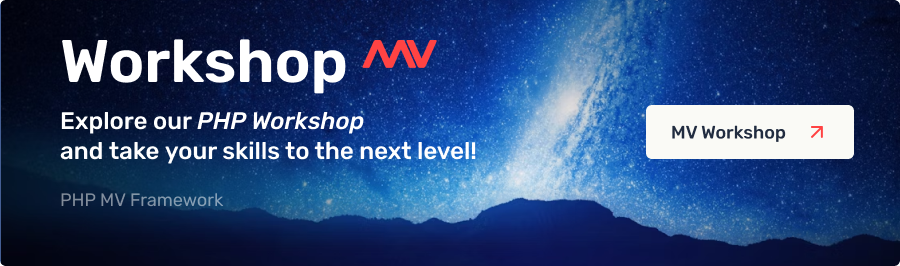