Creating forms
Creating forms in MV has similar principles to creating models. You need to specify a list of fields, and then call methods to display and check the form data.
There are 2 approaches to creating a form:
- Create a form based on a ready model.
- Create a new form manually from the list of fields.
Form from model
Let's assume there is a Questions and Answers model, the site needs to organize the ability for visitors to leave questions, which will then be moderated in the admin panel.
class Faq extends Model
{
protected $name = 'Questions and answers';
protected $model_elements = [
['Active', 'bool', 'active'],
['Name', 'char', 'name', ['required' => true]],
['Date', 'date_time', 'date'],
['Question text', 'text', 'question', ['required' => true]],
['Answer text', 'text', 'answer']
];
public function display()
{
... //Displaying a list of questions and answers
}
}
So, we have a ready-made model, some of whose fields need to be displayed on the site as a form. The fields we need are: 'Name' and 'Question text'. Let's assume that our form is displayed in the views/view-help.php template.
<?
$content = $mv -> pages -> find(['url' => 'help', 'active' => 1]);
$form = new Form('Faq');
$form -> useTokenCSRF();
?>
<h1><? echo $content -> name; ?></h1>
<form method='post'>
<?
echo $form -> display(['name', 'question']);
echo $form -> displayTokenCSRF();
?>
<button>Submit</button>
</form>
Checking the correctness of the form fields and displaying a list of errors.
<?
$form = new Form('Faq');
$form -> useTokenCSRF();
$form -> submit() -> validate();
if($form -> isSubmitted() && $form -> isValid())
{
...
}
?>
<? echo $form -> displayErrors(); ?>
<form method='post'>
...
</form>
After checking the correctness of the fields, we need to send a new question to the database and reload the page to reset the POST data.
if($form -> isSubmitted() && $form -> isValid())
{
$record = $mv -> faq -> getEmptyRecord();
$record -> setValues($form -> all());
$record -> date = I18n::getCurrentDateTime();
$record -> create();
$mv -> reload('?sent');
}
The form can also be built on the basis of a specific record from the model. Such a need arises, for example, to edit a record outside the admin panel. In this case, the second parameter when creating the form should be the id of the desired record from the model, and then the values โโโโof this record will be transferred to the form fields.
//Form from the model and a specific record
$form = new Form('Clients', 52);
$form -> loadRecord();
//You can pass an array of fields that need to be filled from the record data
$form -> loadRecord(['first_name', 'last_name', 'phone', 'email']);
Form without binding to a model
To create a form separate from the model, you need to specify a list of fields. The methods for working with such a form are similar to the previous example.
$fields = [
['Name', 'char', 'name', ['required' => true]],
['Email', 'email', 'email'],
['File', 'file', 'file', ['files_folder' => 'form_files']],
['Message', 'text', 'message', ['required' => true]]
];
$form = new Form($fields);
If the form contains fields of the file, image, multi_images type, then you need to add JavaScript code to the page for buttons for deleting already uploaded files (it is assumed that the JQuery library is connected). This script allows you to delete the selected file and return the field for uploading a new file.
$(document).ready(function()
{
$('form .field-input span.delete').on('click', function()
{
$(this).parents('div.file-params').empty().next().show();
});
});
Previous section
Special methods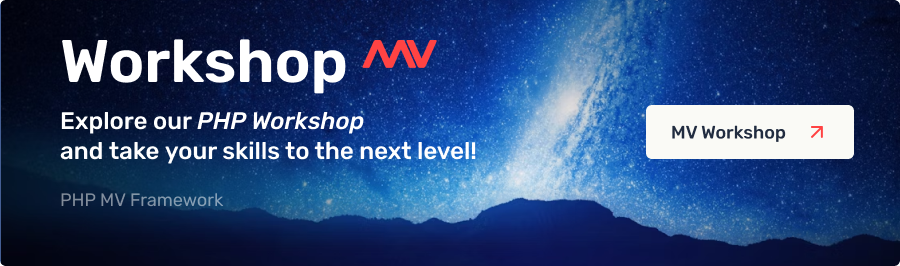