Launching a simple website on MV
This section provides an example of launching a simple website on MV. It assumes the framework installation and launch have already been completed.
Let’s assume there is an HTML template available.
<head>
<title>My application</title>
<link rel='stylesheet' type='text/css' href='/media/css/style.css' />
</head>
<body>
<header>
<ul>
<li class='active'><a href='/about'>About us</a></li>
<li><a href='/services'>Services</a></li>
<li><a href='/contacts'>Contacts</a></li>
</ul>
</header>
<section class='content'>
<h1>Page title</h1>
<p>Page content</p>
</section>
<footer>© Hello world</footer>
</body>
Here, the Pages model will be used, as described in the Preinstalled models section. Models for the site are already created, so we’ll work only with templates from the views folder. More details on routing can be found in the Template principles and MV Object sections.
1. Dividing the template into header and footer
Most websites have repeating top and bottom sections, commonly referred to as header and footer. These sections are separated from the main template into individual files and included at the top and bottom of pages.
In MV, the header and footer are represented by the main-header.php and main-footer.php files in the views folder. Let’s separate the top and bottom sections from our template and save them in these files.
Content of the views/main-header.php file
<head>
<title><? echo $mv -> seo -> title; ?></title>
<meta name='description' content='<? echo $mv -> seo -> description; ?>' />
<meta name='keywords' content='<? echo $mv -> seo -> keywords; ?>' />
<link rel='stylesheet' type='text/css' href='<? echo $mv -> media_path; ?>css/style.css' />
</head>
<body>
<header>
<ul>
<? echo $mv -> pages -> displayMenu(-1); ?>
</ul>
</header>
Here, the displayMenu() method of the Pages model is used to render the menu. It takes the ID of the parent section as a parameter, from which the menu items are displayed. To display items from the root section, set the ID parameter to -1. Additional menu display examples can be found in the Preinstalled models section.
Content of the views/main-footer.php file
<footer>© Hello world</footer>
</body>
2. Main page pemplate
Open the view-index.php file, its initial starting content can be removed.
Add the elements of our template to get the final layout for the views/view-index.php file.
<?
//We search for a row in the database with the contents of the main page
$content = $mv -> pages -> defineCurrentPage($mv -> router);
//If not found, show 404 error
$mv -> display404($content);
//Combine parameters for meta tags
$mv -> seo -> mergeParams($content, 'name');
//We connect the upper part of the template and output the fields from the database
include $mv -> views_path.'main-header.php';
?>
<section class='content'>
<h1><? echo $content -> name; ?></h1>
<? echo $content -> content; ?>
</section>
<?
//We connect the lower part of the template
include $mv -> views_path.'main-footer.php';
?>
3. Standard Inner page template
This template is displayed if the requested page is not the main page and all other templates have been bypassed by the router. Navigating to this template is not a 404 error, though if this page is undefined during URL parsing, the request will move to the 404 error page template.
This template corresponds to the ‘fallback’ route in the config/routes.php file.
Final layout of the views/view-default.php file.
<?
$content = $mv -> pages -> defineCurrentPage($mv -> router);
$mv -> display404($content);
$mv -> seo -> mergeParams($content, 'name');
include $mv -> views_path.'main-header.php';
?>
<section class='content'>
<h1><? echo $content -> name; ?></h1>
<? echo $content -> content; ?>
</section>
<?
include $mv -> views_path.'main-footer.php';
?>
4. 404 error template
If all steps to locate the page in the database have been completed and the page remains undefined, the 404 error template is invoked. MV provides a specific template for this case: views/view-404.php.
Final content of this file for our site.
<?
$content = $mv -> pages -> find(['url' => 'e404']);
$mv -> seo -> mergeParams($content, 'name');
include $mv -> views_path.'main-header.php';
?>
<section class='content'>
<h1><? echo $content -> name; ?></h1>
<? echo $content -> content; ?>
</section>
<?
include $mv -> views_path.'main-footer.php';
?>
5. Site content management
Now that all templates are configured, you can access the administrative section of the site, which is located by default at /adminpanel. In the Page menu section, you can create, edit, and delete pages, which will then be displayed in the menu.
The page with the 'Link' field set to 'index' will be the main page of the site. The content of the 404 error page will be taken from the page with the link field set to 'e404'.
Previous section
Folder structure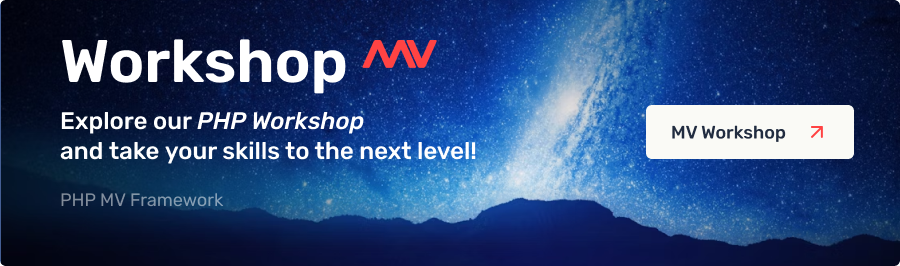