General principles of templates
In MV, templates (views) are php files located in the views folder. These files are responsible for generating HTML code and the appearance of the application. Templates are connected via the routing system. Each requested URL will match one of the templates, even if the page is not found in the database.
All templates must be located in the root views folder, and you can also create nested folders in this folder to group templates.
//Initial list of routes, config/routes.php file
$mvFrontendRoutes = [
'/' => 'view-index.php',
'e404' => 'view-404.php',
'fallback' => 'view-default.php',
'/form' => 'view-form.php'
];
In addition to the basic mandatory routes (index, 404 and fallback), the following types of routes can be created.
- Strict pattern matching.
- A route with an optional part (only one and only at the end).
- A route with one or more variable parts.
- A combination of options 2 and 3.
Below is an example of a config/routes.php file with different types of routes.
$mvFrontendRoutes = [
'/search' => 'view-search.php',
'/pricelist/new' => 'sections/view-price.php',
'/request/?' => 'forms/view-request.php',
'/webhook/paypal' => 'pages/view-pay-confirm.php',
'/news' => 'sections/view-news.php',
'/news/*' => 'sections/view-news-details.php',
'/order/*/*/?' => 'shop/orders/view-check.php',
'/sitemap.xml' => 'seo/sitemap.php'
];
The Router class is responsible for routing and connecting templates, which contains the requested URL. This class stores the original URL, as well as an array of URL parts. The object is described in more detail in the section Router object.
Examples of working with the Router object.
//Getting a full URL
$mv -> router -> getUrl();
//Getting an array of URL parts
$mv -> router -> getUrlParts();
//Getting the first part of the URL
$mv -> router -> getUrlPart(0);
Router operation order
- First, the router checks the URL for a request for the main (index) page of the site.
- If the requested page is not the main page, a search is made for a strict match of the URL to one of the templates (such templates are, for example, /contacts, /form, /register/complete).
- Then, if no strict match is found, a template of the type /contacts/? is searched for with the last optional part.
- Next, a check is made for URL compliance with templates of the type module/* , module/action/*/?, etc.
- If no match for the URL template is found, then the transition to the fallback template occurs.
- If the fallback template does not define the page, then the e404 template (page not found) is called. Also, the 404 template can be called in any other template when it is not possible to identify the page by the passed URL.
Below is a typical method for determining the parameters for searching a page in a database by URL, examples of working with URLs like /event/32 and /event/birthday.
//File config/routes.php
'/event/*' => 'view-event.php'
//File models/events.model.php
class Events extends Model
{
...
public function defineEventPage(Router $router)
{
$url_parts = $router -> getUrlParts();
if(is_numeric($url_parts[1]))
return $this -> find(['id' => $url_parts[1], 'active' => 1]);
else
return $this -> find(['url' => $url_parts[1], 'active' => 1]);
return null;
}
}
//Example of a method call at the beginning of the views/view-event.php file
$event = $mv -> events -> defineEventPage($mv -> router);
$mv -> display404($event);
Previous section
Additional features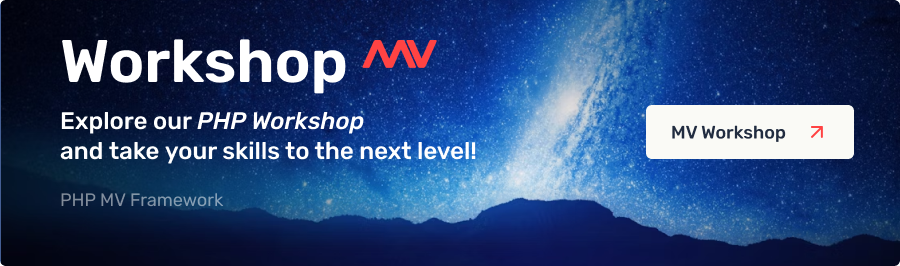